Chat & image generation
Multi-modality is something we have seen more. AI Assistants that are able to generate text, video, images and audio can be super powerful. This tempplate aims to show how you can build an AI Assistant that can both generate text and images. We will be using the newly released Playground v2.
Prerequisites
- A Superagent account and API key
- A Replicate account and API key.
Demo
Setup
We will start by installing the Superagent SDK inside of your project.
Next, we will instantiate a new client.
With our client setup we can now go ahead and create a Replicate
tool and corresponding agent.
If you log in to your Superagent agent account you should see an Agent created. Clicking on it should render an agent similar to the image below:
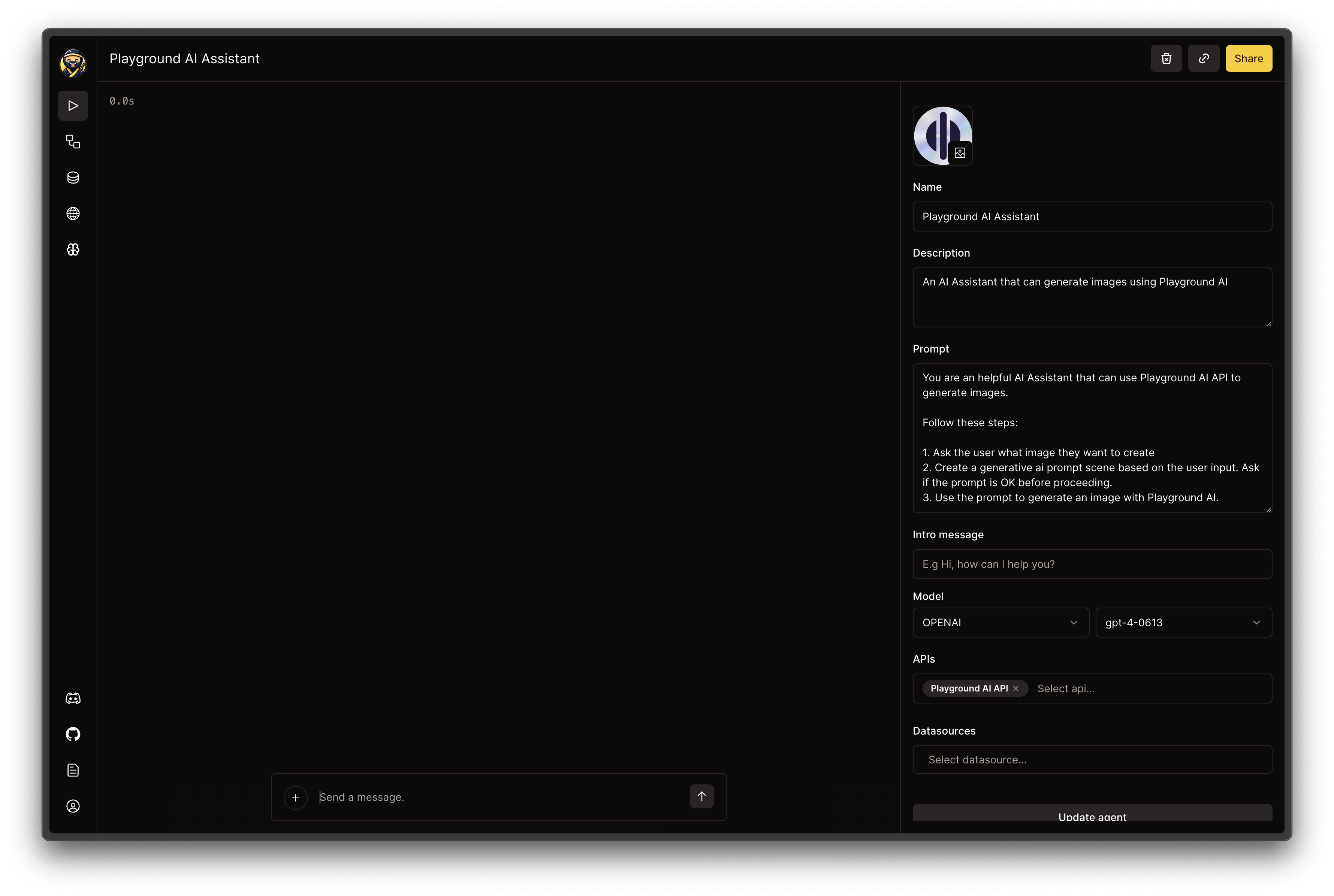
You now have the option to use the SDKs to invoke the agent or use the Superagent UI to start a chat.
Full code
Below you will find the full code for this assistant. You can optionaly use the following NextJS template adapt it.